
Memo
Functions
Function Definition
def functionName():
def functionName(param1, param2, ...):
def functionName(param1="value1", param2="value2", ...):
Allows default values definitions.
All variables within thefunction core are local.
Returned variables usethe keyword returnat the end of the function def.
Control Strutures
for ()...
for x in range(a,b):
dummyList = ['cat', 'dog', 'horse']for animal in dummyList: print len(animal)
If () elif() else...
if (condition1):elif(condition2):else:
While () ...
while (condition2):
Exceptions
try: ... break
except ValueError:
Usefulfunctions
user input
answer = raw_input("what's your name ?")
range
range(5)
range(5,10)
range(1,10,2)
TKinter basics
print number
print type(number)
Files
open a file
idFile = open('myfile.ext', 'w')
write into a file
idFile.write('my text into my file')
close a file
idFile.close()
Variables
CommonDeclarations
no type declaration needed
Integers
number = 5
Floats
number = 3.14159
Strings
littleText = "Go Patriots !"
littleText[3]
Starting index = 0
"Go Patriots !"
littleText[-3]
Negative index means "backward"
"Go Patriots !"
special methods avalaible
string uppercase
dummyStr.upper()
global variable declaration
global fieldGoal
Lists
an powerful array-like structure
special methods avalaible
list length
len(dummyList)
lists concatenation
List1+List2
list repeat
dummyList*5
list element check
x in dummyList
list go through
for each x in dummyList
list append
dummyList.append()
list sort
dummyList.sort()
list search
dummyList.index()
list inversion
dummyList.reverse()
dummyList = [5, 'toto', 2.71]
Dictionnaries
dummyDic1 = {"a":5, "b":11, "c":24}
dummyDic2 = {1:"abc", 2:"def".71}
dummyDic3 = {[1,5]:"metro", [12,5]:"bus", [4,4]:"truck"}
Strings
dummyStr = 'Steelers vs Dolphins'
special methods avalaible
conversion
str(myNumber)
Tuples
Useful variablesfunctions
print number
print type(number)
Operators
power : **
5**2
Logical op.
and
or
not
SyntaxRules
No ";" line ending
Respect indentation
PythonLibraries
User Interface libs
TKinter
website
Scientific computing
Graphics
Multimedia & Gaming
I/O Ports
Pyserial (PC serial port)
Pyparallel (PC parallel port)
Misc.
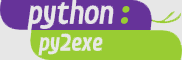
Py2Exe (for Win only)
Oriented-ObjectProgrammation
Class Definition
class myNewClass: def __init__(self,...):
PythonModules
math
from math import *
square root
number = sqrt(2)
trigonometry
number = sin(2)
os
from os import *
change dir
chdir('/tmp')
current working dir
dummyDir = getcwd()
map info
created 2008-07-05 nliebeaux@free.fr
Resources
Docs
French
La doc. de G. Swinnen
English
Websites
Python.org