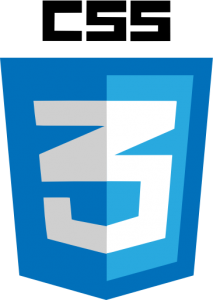
CSS
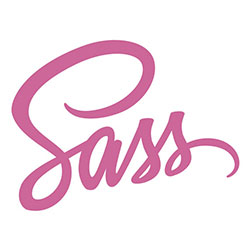
SASS
$variables
_config
good name for variables file
$value-desc--valVariation : property;
good practice
BEM nameing convention
fonts
//font url
$font-url--google
//font stack
//font weight
colors
//descriptive Base Colors
//color usage
$color-primary : $black;
$color-accent : $purple-majesty;
$color-shadow : rgba($black, .2);
text
//font size
$base__font-size : 16px;
$base__line : 24px;
//letter-space
$letter-space : 1px;
images
//path to images
@mixin
use it
like a functions
like a loops
usefull mixins
background-image
ex. usage
generating pseudo elements shapes with @error
@mixin
@content //for pseudo elements
function()
_utilities
file name for functions and mixins
@extend
you can include %extends and rules from other files onto every element
really helpful to make Sass DRY
@extend %extensionName;
@extend .ruleName;
@if variable-exist() {};
@function functionName($parOne, $parTwo : $dafaultValue) {};
usefull functions
//Import if Google Fonts URL is defined
//Calculate pixels to em values
// Call the color palette modifiers
debbuging
@warn
shows warrning in the console
@error
shows error in css output (and browser)
ex.
Sass Maps
like objects in any other language
color
ex.
we can make class first in css, and use it in HTML later
we need @mixin to genetare classes
even more modular
@mixin bg-colors($map) {
@each $theme, $color in $map {
&--#{$theme} {
background-color : $color;
}
}
}
nested maps
pallets of colors
grey & black
how to get it
color : map-get(map-get($palettes, grey) x-grey);
of write a function :)
@function palette($pallete, $shade: 'base') {
@return map-get(map-get($palettes, $pallete), $shade);
}
call it with
color: palette(grey, x-dark);
map-get(map-get($mapName, value) nestedValue);
map-get($mapName, value);
document formating
body
Methodology
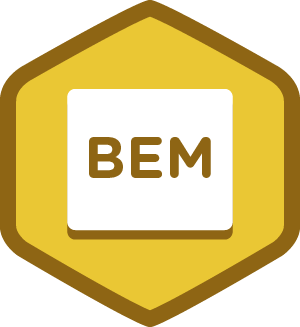
Block Element Modifier
naming convention based on class
help us to write clear and easy to understand CSS
of course not everything can be taken under this nameing convention
ex .site-logo
downsite of BEM approach is lots of classes in html
but
readability is more important
.block__element--modifier
.list {}
.list__item {}
.list__item--block {}
Block = root of the element
highest level of abstraction
Element = child of the root element
Modifier = Styles and Variations
Sass way
BEM @mixin
navigation
ex
with @extend
output 4 classes
headline
ex.
@extension
form
ex.
classes
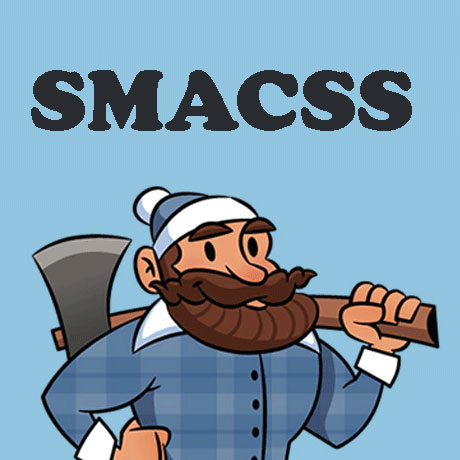
Scalable and Modular Architecture for CSS
to organize and structure CSS on any scale
more like a architecture style guid
divdes project into categories
base
how the elements looks by default
reset styles
use only element selectors
give them default values
no classes and id-s
layout
major sections of the page
header, footer, sidebar, grid
wrappers for other componenets
some developers avoid this category
panel rules for positioning header and footer
@extends
modules
majority of project styles
each module as a separate, reusable component
states
style for element states
:hidden, :active, :expand etc.
themes
diffrent colors and images for diffrent color themes
default category
like for wordpress themes
optionaly
utilities
_mixins
_functions
_config
_helpers
application.scss
@imports
categoryName/_index.scss
from the folders of categories
which contain all the partial files
order of imported parts is important!
sassy - SMACSS based starter for a project
Grid System
nessesery variables
@function to count context
loop to generate calsses
output
mixin to adjust column siblings
we also need to specify a container for grid
usage
class="grid"
center the content
class="grid__col--12"
full width in the grid
Modular Media Queries
variables fro breakpoints
mixin
states at mobile
is displayed at mobile class
collapse at mobile class
styling
height
:<lenght>/%;
with % element is relative to the parent element
px are for precise elements like graphics/icons
width
:<lenght>/%;
with % element is relative to the parent element
px are for precise elements like graphics/icons
max-width
prevents the element from beeing widther than some px values
for good readability on big screens like SamartTV or Mac
making images responsive!
img {max-width: 100%;}
box-sizing
:border-box;
helps us with calculation of size of elements
calculate width and height substracting box model values
it can be universal for whole project
background
it is everyfing exept the margin
everyfing has a transparent background by default
background-color
it is good practice to set the contrast to content color. Even with bg-img in case of problems with displaying it
background-image
: url('path/image.jpg');
it is repaeted horizontaly/verticaly by default
background-size
:<lenght>/% ;
:cover;
fits the image proportionally to the full size of element
best for full background images
relative to the element size
background-repeat
it's :repeat; by default
:repeat-x;
only on x axis
:repeat-y;
only on y axis
:no-reapeat;
background-position
left top corner by default
:x y;
:center center;
= :center;
:right bottom;
:<lenght>/%;
:20% 50%;
it can be write in one line
background-image: #45690 url('path/image.jpg') no-repeat center / cover;
border
ex.
border-radius
: %;
50% is a circle
rounded corners of the element
clip
:rect(0,0,0,0);
list-style
list-style-type
:square/circle/decimal/lover-latin/etc..;
:decimal-leading-zero;
:none;
by default list style is outsite the <li> element
list-style-position
:inside;
to change it
by default list are indented left
padding-left: 0;
to change it
selections
text
line-height
can be unitless
Sass usage for line heights
font-size
font-family
font-style
:italic;
:bold;
text-align
font-weight
letter-spacing
text-decotarion
none
for clearnig list decotarion
class for screen reader text
positioning
Other
comments
/* commented */
structure
basic structure of document
listy
uporządkowane
<ol> <li>...</li> </ol>
domyślnie z numerami, istotna kolejność
CSS list-style-type: ...;
nieuporządkowane
<ul> <li>...</li> </ul>
domyślnie z kropkami, kolejność mniej ważna
<li>...</li>
element listy
listy definicji
<dl>
lista
<dt>
definicja
<dd>
termin
łącza
<a href=""></a>
względne w stosunku do bazowego index.html
../subsite.html
nadrzędny katalog
../../subsite.html
nadrzędny x2 katalog
do poczty
<a href="mailto:email@frank.com>
otwieranie w nowym oknie
<a href="" target="_blank"></a>
przeniesienie w inne miejsce na stronie
zamiast przewijania
<a href="#id elementu"></a>
tak samo można kierować do okteślonych miejsc na innych stronach
<a href="link.html#id elementu"></a>
obrazy
<img src="image.jpg" alt=" wymagany tekst alternatywny" title="tytuł">
<figure><img ...><figcaption>Podpis Obrazu</figcaption></figure>
nowy element HTML5, pozwala na powiązanie obrazu z opisem
tabele
<table></table>
nagłówek tablei
<thead></thead>
<tr></tr>
wiersz
<th scope="row"></th>
nagłówek do rzędów
<th scope="col"></th>
body tabeli
<tbody></tbody>
<td></td>
komórka
łączenie komórek dwóch kolumn w jednym rzędzie
<td colspan=”2”>...</td>
łacznenie komórek dwóch rzędów w kolumnie
<td rowspan=”2”>...</td>
footer tabeli
<tfoot></tfoot>
formularze
<form></form>
<input></input>
type="text" // "password" // "email" // file // submit // image // hidden // date // url // search
maxlenght=23;
określa max liczbę znaków jakie można wpisać
name="identyfikacjaDlaSerwera"
id="dlaLabel"
radio buttons
type="radio"
tylko jedna z opcji możliwa
value="nazwaButtona"
checked="checked"
domyśłnie zaznaczony
checkbox
type="checkbox"
wiele opcji możliwych
<select></select>
<option value="nazwaOpcji"></option>
selected=”selected”
do wyboru domyślnej opcji
multiple
do umożliwienia zaznaczenia kilku opcji
placeholder="wartośćZnikaPoKilknięciu"
method='get' // 'post' // inna metoda
action="..."
link do strony wysyłjącej lub odbierającej informacje
<textarea>Text w textarea</textarea>
obszerny obszar do wpisania textu
<label></label>
etykiety do formularzy
for="IdFormularza"
grupowanie formularzy
<fileset><legend>Nazwa formularza<legend></fileset>
walidacja formularzy
<input type="email" name="poczta" required></input>
<form novalidate></form>
by wyłączyć
inne
<button></button>
semantic
additional meaning like bold, italic etc.
<sup>
indeks górny
<sub>
indeks dolny
</br >
następny akapit
</hr >
linia pomiędzy dwoma akapitami
ważne dla tekstu i czytników ekranowych
<strong>
pogrubienie tekstu, większy nacisk na fragmet tekstu
<em>
domyślnie- kursywa, większy nacisk na znaczenie fragmentu
cytaty
<q>
krótsze cytaty wewnątrz tekstu
<blockquote>
dłuższe cytaty jako osobne paragrafy
udostępniają atrybut cite="..."
dla podania linka do źródła cytatu
<cite></cite>
odwołanie się do książki filmu etc.
sktóry
<abbr></abbr>
atrybut title="..."
pozwala podać znaczenie sktótu
<acronym></acronym>
definicje
<dfn></dfn>
gdy poraz pierwszy wyjaśniamy znaczenie jakiegoś pojęcia
adress
<address></address>
rest
wiadomość do przeglądarki, ze to dokument HTML5
<!DOCTYPE html>
komentarze w HTML
<!-- Nieaktywna treść -->
id=""
calss=""
elementy blokowe
<div></div>
<iframe></iframe>
np. ramka z google maps
seamless
blokuje wyświetlanie pasków przewijania
frameborder
elementy wierszowe
<span></span>
jak div grupuje elementy, ale w jednym wierszu, a nie w całym bloku
można potem dowolnie przerobić fragment tekstu w CSS
<meta />
informacje o stronie zawarte w <head>
może być bez tagu zamykającego
name="nazwaMetaTagu"
description
keywords
oddzielone przecinkami
bez wpływu na pozycje w wyszukiwarce
robots
można wygonić roboty wyszukujace od strony
noindex
mają nie indeksować strony w wyszukiwarkach
nofollow
mają nie indeksować jedynie łączy umieszczonych na stronie
content=" "
wartość metatagu
<meta http-equiv=”pragma”
content=”no-cache” />
zabrania wyszukiwarka zapisywać strony na kompie użytkownika
Subtopic